React-Native
课程目标
- 会使用 RN,了解 RN 同类别的产品,了解移动端的主要技术方案,有一定的跨端开发经验,踩过一些坑;
- 知道如何与 native 进行数据交互,知道 ios 与安卓 jsbridge 实现原理。
- 知道移动端 webview 和基础能力,包括但不限于:webview 资源加载优化方案;webview 池管理、独立进程方案;native 路由等。
- 能够给出完整的前后端对用户体系的整体技术架构设计,满足多业务形态用户体系统一。考虑跨域名、多组织架构、跨端、用户态开放等场景。
知识要点
移动端跨平台开发方案的演进
当前热点
在 2021 JavaScript Rising Star: 链接
- React Native
- lonic
- Expo
- Quasar
- Flipper
- Flutter
演进历史
- Hybrid
- ReactNative (Weex)
- Flutter
- RN + Fabric
端与跨端
- 端:数据获取、状态管理、页面渲染。(FE三板斧)
- 跨端:虚拟机、渲染引擎、原生交互、开发环境。
数据获取
还是老三样:fetch \ axios \ XHR
状态管理
React 中的 state 模式
页面渲染
vDom -> yoga -> iOS / android / DOM APIs -> iOS / android / Web
虚拟机
RN:
- JSC - Objective-c / JS
- 到原生层,用了大量的 bridge
Flutter:
- JIT(dev) + AOT(prod)
- Skia
渲染引擎
yoga
原生交互
Jsbridge
React Native 的原理
React 的设计理念
在运行时开发者能够处理 React JSX 的核心基础其实在于 React 的设计理念,React 将自身能力充分解耦,并提供给社区接入关键环节。这里我们需要先进行一些 React 原理解析。
React 的整体设计理念可以分为三个部分:
- React Core
- React Renderer
- Reconciler
在这里我们需要了解的是:
自定义 renderer — 宿主配置 hostConfig — React reconciler — react core
1 | HostConfig.getPublicInstance |
原理
注册与发布
AppRegistry
是所有 React Native 应用的 JS 入口。应用的根组件应当通过AppRegistry.registerComponent
方法注册自己,然后原生系统才可以加载应用的代码包并且在启动完成之后通过调用AppRegistry.runApplication
来真正运行应用。
1 | AppRegistry.registerComponent(appName, () => App); |
Libraries/ReactNative/AppRegistry.js
1 | registerComponent( |
Libraries/ReactNative/renderApplication.js
1 | function renderApplication<Props: Object>( |
Libraries/Renderer/implementations/ReactNativeRenderer-dev.js
1 | // 22976 |
Renderer
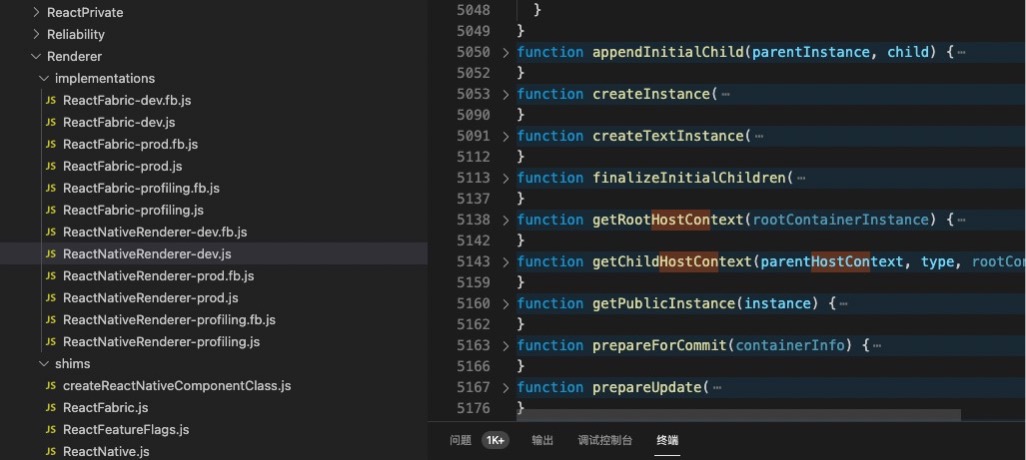
补充知识点
实现⼀个简易 Render
1 | import ReactReconciler from "react-reconciler"; |